In my previous tutorial, I have showed how to implement a simple activity indicator view. This tutorial shows how to extend the SDK’s standard activity indicator by a rounded view which contains the activity indicator view and a text label that looks like the following example:
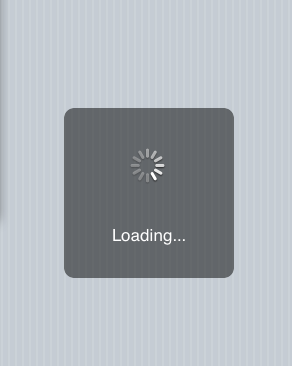
How To
1) First, declare a UIActivityIndicatorView, a UIView and a UILabel instance in the interface class of the view controller in where you want to implement the custom activity indicator.
@interface RootViewController : UIViewController {
UIActivityIndicatorView *activityView;
UIView *loadingView;
UILabel *loadingLabel;
}
@property (nonatomic, retain) UIActivityIndicatorView * activityView;
@property (nonatomic, retain) UIView *loadingView;
@property (nonatomic, retain) UILabel *loadingLabel;
2) Second, synthesize all three variables into the implementation class and add the following code into the viewDidLoad method:
- (void)viewDidLoad {
[super viewDidLoad];
loadingView = [[UIView alloc] initWithFrame:CGRectMake(75, 155, 170, 170)];
loadingView.backgroundColor = [UIColor colorWithRed:0 green:0 blue:0 alpha:0.5];
loadingView.clipsToBounds = YES;
loadingView.layer.cornerRadius = 10.0;
activityView = [[UIActivityIndicatorView alloc] initWithActivityIndicatorStyle:UIActivityIndicatorViewStyleWhiteLarge];
activityView.frame = CGRectMake(65, 40, activityView.bounds.size.width, activityView.bounds.size.height);
[loadingView addSubview:activityView];
loadingLabel = [[UILabel alloc] initWithFrame:CGRectMake(20, 115, 130, 22)];
loadingLabel.backgroundColor = [UIColor clearColor];
loadingLabel.textColor = [UIColor whiteColor];
loadingLabel.adjustsFontSizeToFitWidth = YES;
loadingLabel.textAlignment = UITextAlignmentCenter;
loadingLabel.text = @"Loading...";
[loadingView addSubview:loadingLabel];
[self.view addSubview:loadingView];
[activityView startAnimating];
}
Since I am fan of learing by doing, I will cover the above code on the surface and not in detail. At first, the loading view is initialized and configured with size and rounded corners. It forms the darkend part in which the activity indicator and the text label appears. Next, the activity view is initialized, configured and added as sub view to the loading view. Same holds to the loading label which is also initialized, configured with some parameters and added as sub view to the loading view. At last, the loading view containing the activity indicator view and the loading label is added as sub view to the super view of the view controller (in our case RootViewController).
3) Now start the activity indicator by implementing
[activityView startAnimating];
at the point of the implementation class where the data processing or loading is starting. When the processing or loading job is done, stop the activity indicator animation and remove the activity indicator view from super view by implementing
[activityView stopAnimating];
[loadingView removeFromSuperview];
4) Last but not least, import QuartzCore class into view controller’s implementation class.
#import "QuartzCore/QuartzCore.h"
That’s it! Now, build your app and go to the result showed in the image above 😉